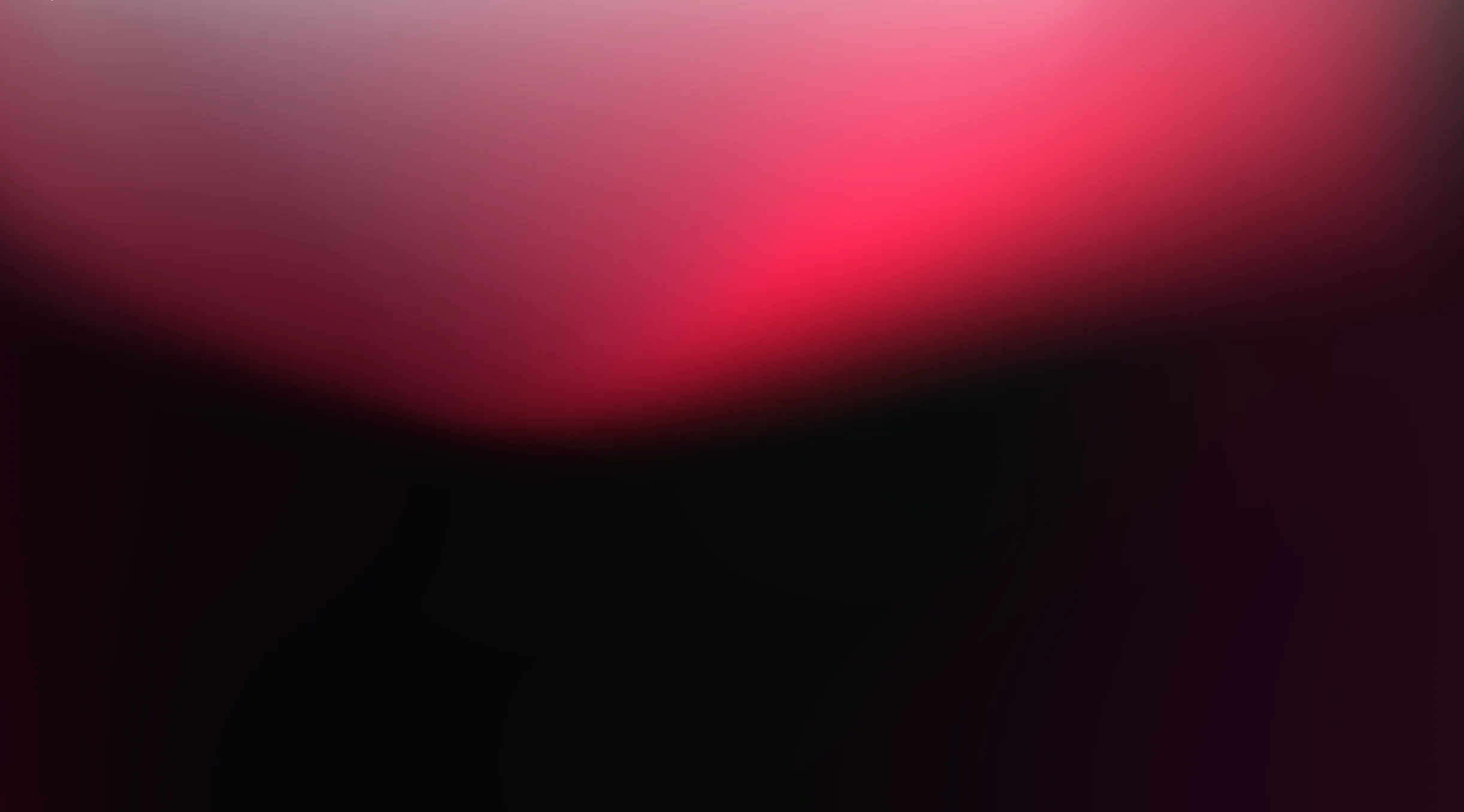
utilities types
Theme Types
v.1.0.0 | SaturnTo facilitate the management of applied tokens across various components, specific types have been created for grouping them:
- CommonStyleType: This grouping of tokens is used to give shape to the containers, being able to apply styles referring to sizes, display, margins, paddings, backgrounds, etc.
- TypographyTypes: This type of tokens is used to give shape to the texts, being able to apply styles referring to sizes, weights, alignments, colours, etc.
- IconTypes: This type of tokens is used to give shape to icons, being able to apply styles referring to size and colours.
- IllustrationTypes: Similar to IconTypes, this type of tokens is used to shape illustrations, being able to apply styles referring to its size.
While all the tokens we will discuss now can be applied directly, they can also be applied for a specific device. In order to apply custom device styles, use the DeviceBreakpointsType enum.
Example
Let's imagine we have the following structure:
type ExampleThemeType = { container?: CommonStyleType; };
We could define the following style in which mobile-specific styles are applied:
const theme: ExampleThemeType = { container: { padding: '10px', background_color: 'red' [DeviceBreakpointsType.MOBILE]: { padding_top: '0px' background_color: 'blue' }, }, };
In this case, the padding and background colour will be applied to all devices, but the padding top and the blue background colour will be applied to mobile only.
CommonStyleType
This specific combination of tokens is used to define the structure and appearance of containers. These tokens allow the application of a wide range of styles that affect the size, layout, margins, padding, backgrounds and other visual aspects of the elements in the interface.
Internally they are grouped into the following subcategories:
- PaddingTypes: tokens associated with paddings.
- BorderTypes: tokens associated with borders.
- BoxShadowTypes: tokens associated with shadows.
- BackgroundTypes: tokens associated with backgrounds.
- MeasuresTypes: tokens associated with sizes.
- DisplayTypes: tokens associated with display.
- MarginTypes: tokens associated with margins.
- PositionTypes: tokens associated with positions.
- PointerTypes: tokens associated with cursors.
- AnimationType: tokens associated with animations.
- WordWrapTypes: tokens associated with line breaks.
Let's look in each subcategory.
PaddingTypes
Token | CSS Property |
---|---|
padding | padding |
padding_left | padding-left |
padding_right | padding-right |
padding_top | padding-top |
padding_bottom | padding-bottom |
BorderTypes
Token | CSS Property |
---|---|
border | border |
border_top | border-top |
border_right | border-right |
border_bottom | border-bottom |
border_left | border-left |
border_radius | border-radius |
border_width | border-width |
border_left_width | border-left-width |
border_right_width | border-right-width |
border_top_width | border-top-width |
border_bottom_width | border-bottom-width |
border_color | border-color |
border_left_color | border-left-color |
border_right_color | border-right-color |
border_top_color | border-top-color |
border_bottom_color | border-bottom-color |
border_top_left_radius | border-top-left-radius |
border_top_right_radius | border-top-right-radius |
border_bottom_left_radius | border-bottom-left-radius |
border_bottom_right_radius | border-bottom-right-radius |
border_style | border-style |
border_left_style | border-left-style |
border_top_style | border-top-style |
border_right_style | border-right-style |
border_bottom_style | border-bottom-style |
outline | outline |
outline_style | outline-style |
outline_color | outline-color |
outline_width | outline-width |
outline_offset | outline-offset |
BoxShadowTypes
Token | CSS Property |
---|---|
box_shadow | box-shadow |
BackgroundTypes
Token | CSS Property |
---|---|
background | background |
background_color | background-color |
background_image | background-image |
background_position | background-position |
background_repeat | background-repeat |
background_size | background-size |
opacity | opacity |
accent_color | accent-color |
MeasuresTypes
Token | CSS Property |
---|---|
box_sizing | box-sizing |
width | width |
min_width | min-width |
max_width | max-width |
height | height |
min_height | min-height |
max_height | max-height |
DisplayTypes
Token | CSS Property |
---|---|
display | display |
visibility | visibility |
overflow | overflow |
overflow_x | overflow-x |
overflow_y | overflow-y |
flex | flex |
flex_direction | flex-direction |
flex_wrap | flex-wrap |
flex_flow | flex-flow |
flex_grow | flex-grow |
flex_shrink | flex-shrink |
flex_basis | flex-basis |
justify_content | justify-content |
align_items | align-items |
align_self | align-self |
align_content | align-content |
order | order |
flex_order | flex-order |
flex_align_self | flex-align-self |
flex_justify_self | flex-justify-self |
flex_align_content | flex-align-content |
flex_align_items | flex-align-items |
gap | gap |
row_gap | row-gap |
column_gap | column-gap |
grid | grid |
grid_area | grid-area |
grid_template | grid-template |
grid_template_areas | grid-template-areas |
grid_template_rows | grid-template-rows |
grid_template_columns | grid-template-columns |
grid_row | grid-row |
grid_row_start | grid-row-start |
grid_row_end | grid-row-end |
grid_column | grid-column |
grid_column_start | grid-column-start |
grid_column_end | grid-column-end |
grid_gap | grid-gap |
grid_row_gap | grid-row-gap |
grid_column_gap | grid-column-gap |
grid_auto_flow | grid-auto-flow |
grid_auto_rows | grid-auto-rows |
grid_auto_columns | grid-auto-columns |
MarginTypes
Token | CSS Property |
---|---|
margin | margin |
margin_left | margin-left |
margin_right | margin-right |
margin_top | margin-top |
margin_bottom | margin-bottom |
PositionTypes
Token | CSS Property |
---|---|
position | position |
top | top |
right | right |
bottom | bottom |
left | left |
float | float |
transform | transform |
transform_style | transform-style |
z_index | z-index |
PointerTypes
Token | CSS Property |
---|---|
cursor | cursor |
pointer_events | pointer-events |
AnimationType
Token | CSS Property |
---|---|
transition | display |
transition_delay | transition-delay |
transition_duration | transition-duration |
transition_property | transition-property |
transition_timing_function | transition-timing-function |
WordWrapTypes
Token | CSS Property |
---|---|
word_break | word-break |
word_wrap | word-wrap |
white_space | white-space |
text_overflow | text-overflow |
TypographyTypes
This specific combination of tokens is used to define the structure and appearance of texts. These tokens allow the application of a wide range of styles that affect the size, weight, alignment, colour and other visual aspects of the text elements.
One of the main tokens to be taken into account is font_variant. This allows to apply a specific variant of the Text component, applying the styles associated with it.
Additionally, the following tokens are available:
Token | CSS Property |
---|---|
font_family | font-family |
font_size | font-size |
font_weight | font-weight |
font_style | font-style |
line_height | line-height |
letter_spacing | letter-spacing |
text_align | text-align |
text_decoration | text-decoration |
text_transform | text-transform |
text_shadow | text-shadow |
text_indent | text-indent |
text_justify | text-justify |
color | color |
overflow | overflow |
IconTypes
This specific combination of tokens is used to define the appearance of the icons. Specifically:
- colour: colour applied to the icon. If the icon already has a colour, it is not necessary to apply this token. Also do not use if the icon has more than one colour.
- width: width of the icon.
- height: height of the icon.
IllustrationTypes
This specific combination of tokens is used to define the size of the illustrations. Specifically:
- width: width of the illustration.
- height: height of the illustration.