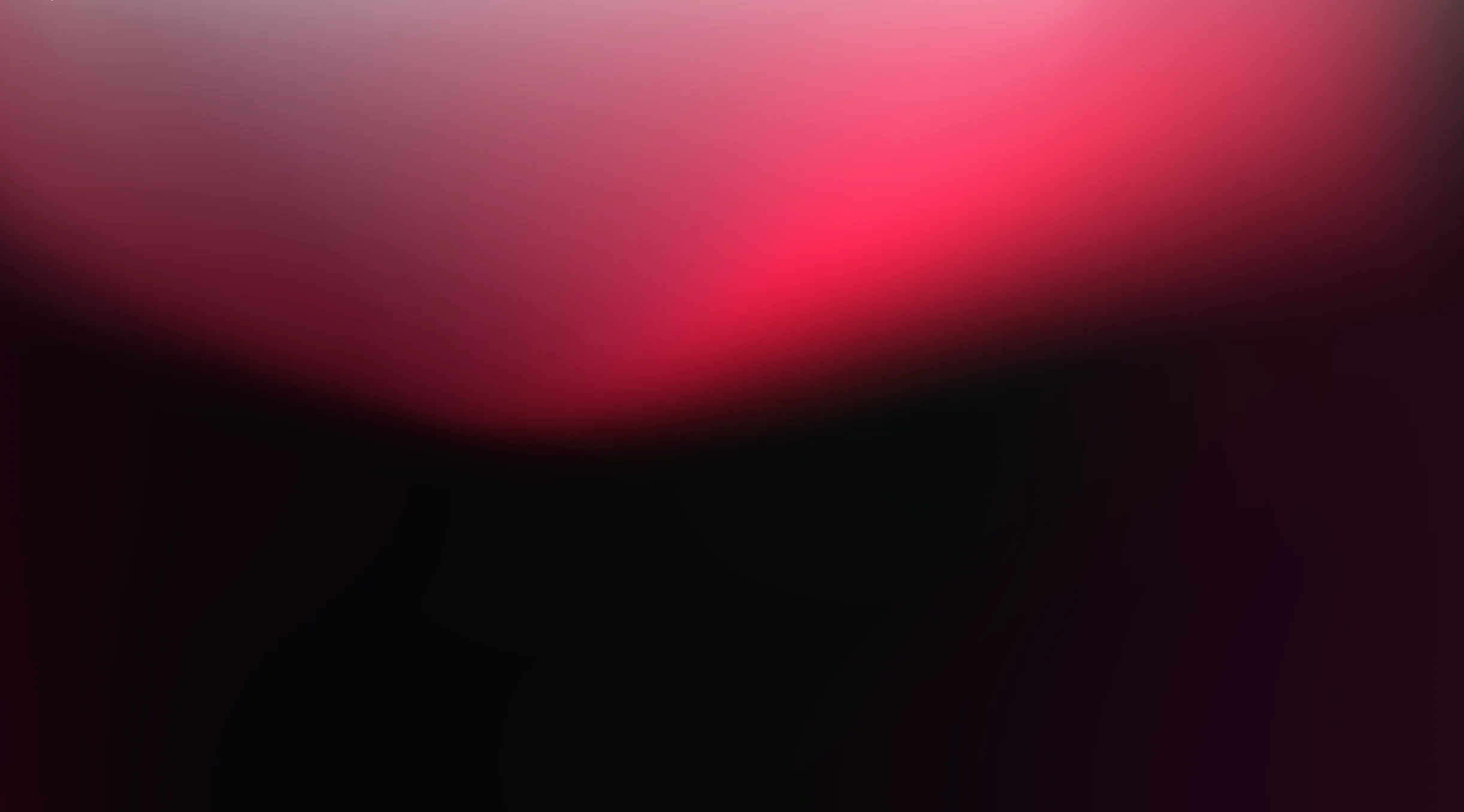
get started
Styling API
v.1.0.0 | SaturnKubit Web Components theming is based on tokens and custom variables.
You can customize the look and feel of the components by overriding the default values of the tokens for the existing component variables, or adding new ones.
Providers
ThemeProvider
The ThemeProvider component is used to set the theme of the components. The next props can be used to set the theme:
- styles: The theme object have the components' styles along with the foundations (colors, borders, ...). These styles are typed objects that the library provides. In case of components' styles like BUTTON_STYLES or TABLE_STYLES, you can find the available tokens and their structure in the inner component documentation.
import { ThemeProvider, KUBIT_STYLES } from '@kubit-ui-web/react-components'; const CUSTOM_STYLES = { ...KUBIT_STYLES, BUTTON_STYLES: { PRIMARY: { DEFAULT: { background_color: 'red', color: 'white', }, }, }, }; const App = () => { <ThemeProvider styles={CUSTOM_STYLES}> <Button variant="PRIMARY">Primary</Button> </ThemeProvider>; };
- customGlobalStyles: Prop that allows adding custom global styles to the theme. These styles are applied to the root element of the application. Among others, it includes font and focus styles.
import { css } from 'styled-components'; const CUSTOMGLOBAL_STYLES = css` *, *::before, *::after { box-sizing: border-box; } `; <ThemeProvider customGlobalStyles={CUSTOMGLOBAL_STYLES} />;
- themeInformation: Prop that allows adding information about the theme. For example, a name or description.
const themeInformation = { name: 'My theme', description: 'This is my theme', }; <ThemeProvider themeInformation={themeInformation} />;
- genericComponents: Prop that allows configuring the generic components of the project. Example of this kind of components are links. In some project you would expect to be an a components, but maybe in other project, a Link from react-router.
const Link = React.forwardRef(function Link(props: IGenericLink, ref: unknown) { return <a href={props.url}>{props.children}</a>; }); const genericComponents = { Link: Link, }; <ThemeProvider genericComponents={genericComponents} />;
UtilsProvider
Use this provider to set some utils and extra configurations that the library use in the projects.
import { ThemeProvider, UtilsProvider } from '@kubit-ui-web/react-components'; <UtilsProvider> <ThemeProvider>{children}</ThemeProvider> </UtilsProvider>;
If you are using KubitThemeProvider, you don't need to use UtilsProvider. KubitThemeProvider already has UtilsProvider inside.
utilsProvider has the following props:
- assets: Configuration of the assets of the projects. If the assets (images, icons, illustrations) are hosted on a CDN, you can configure the base url here.
- baseHost: Base url of the assets.
- iconsBaseHost: Base url of the icons.
- illustrationsBaseHost: Base url of the illustrations.
const customAssets = { baseHost: 'https://assets.myproject.com', iconsBaseHost: 'https://assets.myproject.com/icons', illustrationsBaseHost: 'https://assets.myproject.com/illustrations', }; <UtilsProvider assets={customAssets} />;
- dateHelpers: Configuration of the date helpers. If you want to use components like InputDate, you need to configure the date helpers. We recommend to use date-fns library or dayjs. The props that you need to configure are:
- isAfter: Function to know if date is after other date.
- isBefore: Function to know if date is before other date.
- isDatesEqual: Function to know if two dates are equal.
- getAddDays: Function to add days to a date.
- getAddMonths: Function to add months to a date.
- getAddYears: Function to add years to a date.
- getSubDays: Functon to substract days to a date.
- getSubMonths: Function to substract months to a date.
- getSubYears: Function to substract years to a date.
- getAllMonthName: Function to get all month names.
- getAllWeekdayName: Function to get all weekday names.
import { UtilsProvider } from '@kubit-ui-web/react-components'; const dateHelpers = { isAfter: (date1: Date, date2: Date) => { return date1 > date2; }, } <UtilsProvider dateHelpers={dateHelpers} />
The functions to format and transform dates must be included in formatDate and transformDate props:
- formatDate: Function to format a date.
- transformDate: Function to parse a date.
import { UtilsProvider } from '@kubit-ui-web/react-components'; const formatDate = (date: Date, format: string) => { return format(date, format); }; const transformDate = (date: Date, format: string) => { return parse(date, format); }; <UtilsProvider formatDate={formatDate} transformDate={transformDate} />;
Remember that you need to configure all these functions to use the date components.
Foundations
The foundations are the base of the theme, by default they are provided by kubit. These are the colors, typography, spacing, etc. that are used to style the components.
These values are declared in the theme, but you can create your own foundations. Here is a fundations example:
const FOUNDATIONS = { COLORS: { primary: '#000000', secondary: '#FFFFFF', }, SPACINGS: { small: '8px', medium: '16px', large: '24px', }, };
For more information, visit the specific section visiting Foundations
Breakpoints
Breakpoints are the different screen sizes of the application. You can configure the breakpoints on the theme. The breakpoints are used to configure the responsive styles of the components. You can create your own breakpoints.
This format is used to configure the breakpoints:
type BREAKPOINTS { ZERO: number, S: number, M: number, L: number, XL: number, };
For more information, check the specific section visiting Breakpoints
Variants
Components have variants, they represents different styles of the same component. For example, the button component has a PRIMARY and a SECONDARY variant. You can change the variant of a component by setting the variant prop.
Variants are declared in the theme and you can create your own extending the default theme as explained before. Here is an example of a theme with two variants for the button component:
enum ButtonVariants { PRIMARY = 'PRIMARY', SECONDARY = 'SECONDARY', }
Tokens
Tokens are the building blocks of the theme. They are used to style specific part of a component as CSS custom properties. You can override the default values of the tokens to change the look and feel of the components.
The name and structure of the tokens for each component are specify in the inner component documentation.
Let's see an example for the button component, in which you would like to add a new variant.
First, you define the new variant in an enum:
enum InnerButtonVariants { NEW_VARIANT = 'NEW_VARIANT', }
Then, you can add the tokens for the new variant:
const INNER_BUTTON_STYLES = { [InnerButtonVariants.NEW_VARIANT]: { DEFAULT: { background_color: FOUNDATIONS.COLORS.PRIMARY, }, HOVER: { background_color: FOUNDATIONS.COLORS.SECONDARY, }, }, };
Finally, you could extend the kubit theme as explained before in the ThemeProvider section, and use the new variant for the button component.
Components can configure this tokens for all type of breakpoints (mobile, tablet and desktop). Breakpoints can be configured on the theme. You can find more information about breakpoints on the Breakpoints section.